For those people who are searching for XMLSocket function to work properly, I will tell you step by step on how to create PHP socket server to support XMLSocket function in Flash, and also how to host your crossdomain.xml so it can be use by the Flash 9 loadPolicyFile function.
Step 1:
Since Flash 9 do not support loading the crossdomain.xml file directly by url, we must first create a socket to host the crossdomain.xml.
PHP (policySocketServer.php):
#!/usr/bin/php -q
<?php
error_reporting(E_ALL);
set_time_limit(0);
ob_implicit_flush();
function __autoload ($className)
{
$fileName = "Classes/".str_replace('_', DIRECTORY_SEPARATOR, $className) . '.php';
$status = (@include_once $fileName);
if ($status === false) {
eval(sprintf('class %s {func' . 'tion __construct(){throw new Project_Exception_AutoLoad("%s");}}', $className, $className));
}
}
$ip = '127.0.0.1'; //change it to your webserver path
$port = 9996; //any port no you wish and it must be greater than 1024
//new SocketServer($ip, $port);
try{
$mySocketServer = new SocketServer($ip, $port);
} catch (Project_Exception_AutoLoad $e) {
//echo "FATAL ERROR: CAN'T FIND SOCKET SERVER CLASS.";
}
?>
Create a folder named Classes, and inside the folder create Logger.php and SocketServer.php
PHP (Logger.php):
<?php
class Logger
{
private static $instance;
public static function getInstance()
{
if(!isset($instance))
$instance = new Logger();
return $instance;
}
function __construct()
{
}
function log($message)
{
//echo "[".date('Y-m-d')."] ".$message."\n";
}
}
?>
PHP (SocketServer.php):
<?php
class SocketServer
{
var $ip;
var $port;
var $masterSocket;
var $logger;
private $currentSockets;
function __construct($ip, $port)
{
$this->ip = $ip;
$this->port = $port;
$this->logger = Logger::getInstance();
$this->initSocket();
$this->currentSockets = array();
$this->currentSockets[] = $this->masterSocket;
$this->listenToSockets();
}
private function initSocket()
{
//---- Start Socket creation for PHP 5 Socket Server -------------------------------------
if (($this->masterSocket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP)) < 0)
{
$this->logger->log("[SocketServer] "."socket_create() failed, reason: " . socket_strerror($this->masterSocket));
}
socket_set_option($this->masterSocket, SOL_SOCKET,SO_REUSEADDR, 1);
if (($ret = socket_bind($this->masterSocket, $this->ip, $this->port)) < 0) {
$this->logger->log("[SocketServer] "."socket_bind() failed, reason: " . socket_strerror($ret));
}
if (($ret = socket_listen($this->masterSocket, 5)) < 0) {
$this->logger->log("[SocketServer] "."socket_listen() failed, reason: " . socket_strerror($ret));
}
}
private function parseRequest($socket, $data)
{
if(substr($data,0, 22) == "<policy-file-request/>")
{
//echo "POLICY FILE REQUEST\n";
$crossFile = file("http://localhost/crossdomain.xml"); //put your domain url where you uploaded the crossdomain.xml, it can also be any online webserver path
$crossFile = join('',$crossFile);
$this->sendMessage(array($socket),$crossFile);
return;
}
}
public function sendMessage($sockets, $message)
{
$message .= "\0";
if(!is_array($sockets))
$sockets = array($sockets);
foreach($sockets as $socket)
{
if($socket === NULL)
continue;
socket_write($socket, $message, strlen($message));
//$this->logger->log("[SocketServer] Wrote : ".$message." to ".$socket);
}
}
private function listenToSockets()
{
//---- Create Persistent Loop to continuously handle incoming socket messages ---------------------
while (true) {
$changed_sockets = $this->currentSockets;
$num_changed_sockets = socket_select($changed_sockets, $write = NULL, $except = NULL, NULL);
foreach($changed_sockets as $socket)
{
if ($socket == $this->masterSocket) {
if (($client = socket_accept($this->masterSocket)) < 0) {
$this->logger->log("[SocketServer] "."socket_accept() failed: reason: " . socket_strerror($socket));
continue;
} else {
// NEW CLIENT HAS CONNECTED
$this->currentSockets[] = $client;
socket_getpeername($client, $newClientAddress);
$this->logger->log("[SocketServer] "."NEW CLIENT ".$client." [IP: ".$newClientAddress."]");
}
} else {
$bytes = socket_recv($socket, $buffer, 4096, 0);
if ($bytes == 0) {
// CLIENT HAS DISCONNECTED
$this->logger->log("[SocketServer] "."REMOVING CLIENT ".$socket);
$index = array_search($socket, $this->currentSockets);
// Remove Socket from List
unset($this->currentSockets[$index]);
socket_close($socket);
}else{
// CLIENT HAS SENT DATA
$this->parseRequest($socket, $buffer);
}
}
}
}
}
}
?>
Create the crossdomain.xml file and put it in your webserver root:
XML (crossdomain.xml):
<cross-domain-policy>
<allow-access-from domain="*" to-ports="*"/>
</cross-domain-policy>
Step 2 (if you're running on local server):
Create a batch file in your desktop named runPolicyFile.bat:
Bat (runPolicyFile.bat):
c:/wamp/bin/php/php5.2.5/php.exe -q c:/wamp/www/policySocketServer.php
Click the batch file to run the script (make sure you have your webserver is up and running).
Alternative way is to run the policySocketServer.php straight away in your web browser.
Step 3:
In your flash file, include this script:
System.security.allowDomain("*");
System.security.loadPolicyFile("xmlsocket://127.0.0.1:9996"); //your webserver path and the port number you choose
You're done
You should be able to see that your XMLSocket function working now in the web browser :)
Check out the demo here . I have successfully implement PURE PHP SOCKET SERVER to build this chat application in Flash through XMLSocket function.
Any comment appreciated..
Friday, September 26, 2008
Thursday, September 25, 2008
Flash Interval / timer function
code:
interval = function() {
//do your things here
clearInterval(intervalInt);
}
var intervalInt = setInterval(interval, 1000);
*1000 refers to 1000ms = 1s
interval = function() {
//do your things here
clearInterval(intervalInt);
}
var intervalInt = setInterval(interval, 1000);
*1000 refers to 1000ms = 1s
Wednesday, September 24, 2008
Tuesday, September 23, 2008
PHP list files in directory
code:
// open this directory
$myDirectory = opendir(".");
// get each entry
while($entryName = readdir($myDirectory)) {
$dirArray[] = $entryName;
}
// close directory
closedir($myDirectory);
// count elements in array
$indexCount = count($dirArray);
//Print ("$indexCount files<br>\n");
// sort 'em
sort($dirArray);
// print 'em
print("<TABLE border=1 cellpadding=5 cellspacing=0 class=whitelinks>\n");
print("<TR><TH>Filename</TH><th>Filetype</th><th>Filesize</th><th>Created</th></TR>\n");
// loop through the array of files and print them all
for($index=0; $index < $indexCount; $index++) {
$checkExt = explode(".", $dirArray[$index]);
if (substr("$dirArray[$index]", 0, 1) != "." && $checkExt[count($checkExt)-1] != "php"){ // don't list hidden and php files
print("<TR><TD><a href=\"$dirArray[$index]\">$dirArray[$index]</a></td>");
print("<td>");
print(filetype($dirArray[$index]));
print("</td>");
print("<td>");
print(filesize($dirArray[$index]));
print("</td>");
print("<td>");
print(date("F d Y H:i:s.", filectime($dirArray[$index])));
print("</td>");
print("</TR>\n");
}
}
print("</TABLE>\n");
clearstatcache();
// open this directory
$myDirectory = opendir(".");
// get each entry
while($entryName = readdir($myDirectory)) {
$dirArray[] = $entryName;
}
// close directory
closedir($myDirectory);
// count elements in array
$indexCount = count($dirArray);
//Print ("$indexCount files<br>\n");
// sort 'em
sort($dirArray);
// print 'em
print("<TABLE border=1 cellpadding=5 cellspacing=0 class=whitelinks>\n");
print("<TR><TH>Filename</TH><th>Filetype</th><th>Filesize</th><th>Created</th></TR>\n");
// loop through the array of files and print them all
for($index=0; $index < $indexCount; $index++) {
$checkExt = explode(".", $dirArray[$index]);
if (substr("$dirArray[$index]", 0, 1) != "." && $checkExt[count($checkExt)-1] != "php"){ // don't list hidden and php files
print("<TR><TD><a href=\"$dirArray[$index]\">$dirArray[$index]</a></td>");
print("<td>");
print(filetype($dirArray[$index]));
print("</td>");
print("<td>");
print(filesize($dirArray[$index]));
print("</td>");
print("<td>");
print(date("F d Y H:i:s.", filectime($dirArray[$index])));
print("</td>");
print("</TR>\n");
}
}
print("</TABLE>\n");
clearstatcache();
Javascript for removing spaces in string
code:
<script type="text/javascript">
function removeSpace(obj) {
var tstring = "";
string = '' + document.getElementById(obj).value;
splitstring = string.split(" ");
for(g = 0; g < splitstring.length; g++){
tstring += splitstring[g];
document.getElementById(obj).value = tstring;
}
}
</script>
sample (put in your html code):
<script type="text/javascript">
function removeSpace(obj) {
var tstring = "";
string = '' + document.getElementById(obj).value;
splitstring = string.split(" ");
for(g = 0; g < splitstring.length; g++){
tstring += splitstring[g];
document.getElementById(obj).value = tstring;
}
}
</script>
<textarea name="tb" id="tb" cols="60" rows="10" onKeyUp="removeSpace('tb')"></textarea>
*Type anything inside the textArea
<script type="text/javascript">
function removeSpace(obj) {
var tstring = "";
string = '' + document.getElementById(obj).value;
splitstring = string.split(" ");
for(g = 0; g < splitstring.length; g++){
tstring += splitstring[g];
document.getElementById(obj).value = tstring;
}
}
</script>
sample (put in your html code):
<script type="text/javascript">
function removeSpace(obj) {
var tstring = "";
string = '' + document.getElementById(obj).value;
splitstring = string.split(" ");
for(g = 0; g < splitstring.length; g++){
tstring += splitstring[g];
document.getElementById(obj).value = tstring;
}
}
</script>
<textarea name="tb" id="tb" cols="60" rows="10" onKeyUp="removeSpace('tb')"></textarea>
*Type anything inside the textArea
Monday, September 22, 2008
How to activate flash object without clicking in Internet Explorer (IE)
1. Create a file, flashIEfix.js and put this code in it:
objects = document.getElementsByTagName("object");
for (var i = 0; i < objects.length; i++)
{
objects[i].outerHTML = objects[i].outerHTML;
}
2. Put this script to your flash html page:
<script type="text/javascript" src="flashIEfix.js"></script>
objects = document.getElementsByTagName("object");
for (var i = 0; i < objects.length; i++)
{
objects[i].outerHTML = objects[i].outerHTML;
}
2. Put this script to your flash html page:
<script type="text/javascript" src="flashIEfix.js"></script>
Sunday, September 21, 2008
Flash transform color
code:
colorChange = new Color(MovieClip);
myColorTransform = new Object();
// Set the values for myColorTransform
myColorTransform = { ra: _global.Rchange, rb: _global.Rchange, ga: _global.Gchange, gb: _global.Gchange, ba: _global.Bchange, bb: _global.Bchange, aa: Alpha, ab: Alpha};
colorChange.setTransform(myColorTransform);
variable: MovieClip
colorChange = new Color(MovieClip);
myColorTransform = new Object();
// Set the values for myColorTransform
myColorTransform = { ra: _global.Rchange, rb: _global.Rchange, ga: _global.Gchange, gb: _global.Gchange, ba: _global.Bchange, bb: _global.Bchange, aa: Alpha, ab: Alpha};
colorChange.setTransform(myColorTransform);
variable: MovieClip
Saturday, September 20, 2008
How to resize image using only PHP script
Here is the very small code sample that will resize images as they are uploaded from a web form. Please read the comments in detail. They explain exactly what is going on, and where you should make changes for the destination directory and output sizes.
This script only supports the JPG file format. The compression algorithm for GIF images is protected by a restrictive licensing agreement that prevents the developers of PHP from including support for GIF images in the base platform.
Part I: The HTML Form
<form action="upload.php" method="post" enctype="multipart/form-data" >
<input type="file" name="uploadfile"/>
<input type="submit"/>
</form>
Part II: Getting at the file, resizing the image, and saving to the server. (upload.php)
<?php
// This is the temporary file created by PHP
$uploadedfile = $_FILES['uploadfile']['tmp_name'];
// Create an Image from it so we can do the resize
$src = imagecreatefromjpeg($uploadedfile);
// Capture the original size of the uploaded image
list($width,$height)=getimagesize($uploadedfile);
// For our purposes, I have resized the image to be
// 600 pixels wide, and maintain the original aspect
// ratio. This prevents the image from being "stretched"
// or "squashed". If you prefer some max width other than
// 600, simply change the $newwidth variable
$newwidth=600;
$newheight=($height/$width)*600;
$tmp=imagecreatetruecolor($newwidth,$newheight);
// this line actually does the image resizing, copying from the original
// image into the $tmp image
imagecopyresampled($tmp,$src,0,0,0,0,$newwidth,$newheight,$width,$height);
// now write the resized image to disk. I have assumed that you want the
// resized, uploaded image file to reside in the ./images subdirectory.
$filename = "images/". $_FILES['uploadfile']['name'];
imagejpeg($tmp,$filename,100);
imagedestroy($src);
imagedestroy($tmp); // NOTE: PHP will clean up the temp file it created when the request
// has completed.
?>
This script only supports the JPG file format. The compression algorithm for GIF images is protected by a restrictive licensing agreement that prevents the developers of PHP from including support for GIF images in the base platform.
Part I: The HTML Form
<form action="upload.php" method="post" enctype="multipart/form-data" >
<input type="file" name="uploadfile"/>
<input type="submit"/>
</form>
Part II: Getting at the file, resizing the image, and saving to the server. (upload.php)
<?php
// This is the temporary file created by PHP
$uploadedfile = $_FILES['uploadfile']['tmp_name'];
// Create an Image from it so we can do the resize
$src = imagecreatefromjpeg($uploadedfile);
// Capture the original size of the uploaded image
list($width,$height)=getimagesize($uploadedfile);
// For our purposes, I have resized the image to be
// 600 pixels wide, and maintain the original aspect
// ratio. This prevents the image from being "stretched"
// or "squashed". If you prefer some max width other than
// 600, simply change the $newwidth variable
$newwidth=600;
$newheight=($height/$width)*600;
$tmp=imagecreatetruecolor($newwidth,$newheight);
// this line actually does the image resizing, copying from the original
// image into the $tmp image
imagecopyresampled($tmp,$src,0,0,0,0,$newwidth,$newheight,$width,$height);
// now write the resized image to disk. I have assumed that you want the
// resized, uploaded image file to reside in the ./images subdirectory.
$filename = "images/". $_FILES['uploadfile']['name'];
imagejpeg($tmp,$filename,100);
imagedestroy($src);
imagedestroy($tmp); // NOTE: PHP will clean up the temp file it created when the request
// has completed.
?>
Flash movieClip drag
this.onPress = function() {
startDrag(this);
}
this.onRelease = function() {
stopDrag();
}
this.onReleaseOutside = function() {
stopDrag();
}
startDrag(this);
}
this.onRelease = function() {
stopDrag();
}
this.onReleaseOutside = function() {
stopDrag();
}
Flash pixel collision detection
The CollisionDetection class is really simple to work with, there is a single static method called checkForCollision with four parameters:
checkForCollision(movieClip1,movieClip2,alphaTolerance);
movieClip1, movieClip2 - The MovieClip instances to check for collision.
alphaTolerance - a number from 0 to 255 that specifies the transparency tolerance when testing the collision. A higher number will increase the tolerance (ie. allow more transparent parts of the MovieClip to register collisions). Defaults to 255.
Returns a Rectangle object specifying the intersection of the two MovieClips in the _root coordinate space, or null if they do not intersect.
code (CollisionDetection.as):
import flash.display.BitmapData;
import flash.geom.ColorTransform;
import flash.geom.Matrix;
import flash.geom.Rectangle;
class CollisionDetection {
static public function checkForCollision(p_clip1:MovieClip,p_clip2:MovieClip,p_alphaTolerance:Number):Rectangle {
// set up default params:
if (p_alphaTolerance == undefined) { p_alphaTolerance = 255; }
// get bounds:
var bounds1:Object = p_clip1.getBounds(_root);
var bounds2:Object = p_clip2.getBounds(_root);
// rule out anything that we know can't collide:
if (((bounds1.xMax < bounds2.xMin) || (bounds2.xMax < bounds1.xMin)) || ((bounds1.yMax < bounds2.yMin) || (bounds2.yMax < bounds1.yMin)) ) {
return null;
}
// determine test area boundaries:
var bounds:Object = {};
bounds.xMin = Math.max(bounds1.xMin,bounds2.xMin);
bounds.xMax = Math.min(bounds1.xMax,bounds2.xMax);
bounds.yMin = Math.max(bounds1.yMin,bounds2.yMin);
bounds.yMax = Math.min(bounds1.yMax,bounds2.yMax);
// set up the image to use:
var img:BitmapData = new BitmapData(bounds.xMax-bounds.xMin,bounds.yMax-bounds.yMin,false);
// draw in the first image:
var mat:Matrix = p_clip1.transform.concatenatedMatrix;
mat.tx -= bounds.xMin;
mat.ty -= bounds.yMin;
img.draw(p_clip1,mat, new ColorTransform(1,1,1,1,255,-255,-255,p_alphaTolerance));
// overlay the second image:
mat = p_clip2.transform.concatenatedMatrix;
mat.tx -= bounds.xMin;
mat.ty -= bounds.yMin;
img.draw(p_clip2,mat, new ColorTransform(1,1,1,1,255,255,255,p_alphaTolerance),"difference");
// find the intersection:
var intersection:Rectangle = img.getColorBoundsRect(0xFFFFFFFF,0xFF00FFFF);
// if there is no intersection, return null:
if (intersection.width == 0) { return null; }
// adjust the intersection to account for the bounds:
intersection.x += bounds.xMin;
intersection.y += bounds.yMin;
return intersection;
}
}
------------------------------------------------------------------------------------
code (in Flash):
import CollisionDetection;
import flash.geom.Rectangle;
createEmptyMovieClip("drawin",100);
drawin.clear();
// check for collisions:
var collisionRect:Rectangle = CollisionDetection.checkForCollision(mc1,mc2,120);
// if there is a collision, draw the intersection:
if (collisionRect) { with (drawin) {
beginFill(0x00FF00,20);
lineStyle(0,0x00FF00,40);
moveTo(collisionRect.x,collisionRect.y);
lineTo(collisionRect.x+collisionRect.width,collisionRect.y);
lineTo(collisionRect.x+collisionRect.width,collisionRect.y+collisionRect.height);
lineTo(collisionRect.x,collisionRect.y+collisionRect.height);
lineTo(collisionRect.x,collisionRect.y);
}}
if (collisionRect) {
//do your stuff
}
------------------------------------------------------------------------------------
Click here to download. It includes two simple demos to help you get started with it.
Flash local connection
code (outgoing msg):
var outgoing_lc:LocalConnection = new LocalConnection();
outgoing_lc.send("sessionName", "methodToExecute", "messageToSend");
code (incoming msg listen):
var incoming_lc:LocalConnection = new LocalConnection();
incoming_lc.connect("sessionName");
incoming_lc.methodToExecute = function(param:String):Void {
if (param == "messageToSend") {
//do your things
}
}
var outgoing_lc:LocalConnection = new LocalConnection();
outgoing_lc.send("sessionName", "methodToExecute", "messageToSend");
code (incoming msg listen):
var incoming_lc:LocalConnection = new LocalConnection();
incoming_lc.connect("sessionName");
incoming_lc.methodToExecute = function(param:String):Void {
if (param == "messageToSend") {
//do your things
}
}
Friday, September 19, 2008
Flash load XML from php
code (in flash):
//set array
var company_name:Array = new Array();
var client_id:Array = new Array();
var ClientXML:XML = new XML();
ClientXML.ignoreWhite = true;
var height = 0;
ClientXML.onLoad = function() {
var nodes = this.firstChild.childNodes;
for (i=0;i<nodes.length;i++) {
movname="MovieClip_" + i;
company_name.push(nodes[i].firstChild.nodeValue);
client_id.push(nodes[i].attributes.id);
attachMovie("MovieClip_", movName, i);
eval("MovieClip_" + movName).company_name_tb.text = company_name[i];
eval("MovieClip_" + movName).clientID_tb.text = client_id[i];
eval("MovieClip_" + movName)._y = height;
height += eval("MovieClip_" + movName)._height;
}
}
ClientXML.load("clientflash.php");
-------------------------------------------------------------------------------------
code (clientflash.php - assuming you have the database connection, $client_listRS):
<?php
echo "<?xml version=\"1.0\"?>\n";
echo "<client>\n";
do {
echo "<company id="" . $row_client_listRS[" client_id=""><![CDATA[" . $row_client_listRS['company_name'] . "]]></company>\n";
} while ($row_client_listRS = mysql_fetch_assoc($client_listRS));
echo "</client>\n";
?>
//set array
var company_name:Array = new Array();
var client_id:Array = new Array();
var ClientXML:XML = new XML();
ClientXML.ignoreWhite = true;
var height = 0;
ClientXML.onLoad = function() {
var nodes = this.firstChild.childNodes;
for (i=0;i<nodes.length;i++) {
movname="MovieClip_" + i;
company_name.push(nodes[i].firstChild.nodeValue);
client_id.push(nodes[i].attributes.id);
attachMovie("MovieClip_", movName, i);
eval("MovieClip_" + movName).company_name_tb.text = company_name[i];
eval("MovieClip_" + movName).clientID_tb.text = client_id[i];
eval("MovieClip_" + movName)._y = height;
height += eval("MovieClip_" + movName)._height;
}
}
ClientXML.load("clientflash.php");
-------------------------------------------------------------------------------------
code (clientflash.php - assuming you have the database connection, $client_listRS):
<?php
echo "<?xml version=\"1.0\"?>\n";
echo "<client>\n";
do {
echo "<company id="" . $row_client_listRS[" client_id=""><![CDATA[" . $row_client_listRS['company_name'] . "]]></company>\n";
} while ($row_client_listRS = mysql_fetch_assoc($client_listRS));
echo "</client>\n";
?>
Flash create line art using actionScript
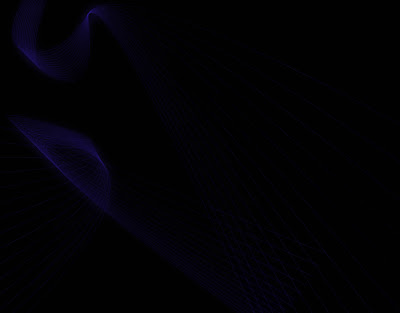
code:
//top line---------------------------------------------------------------
h=0;
while (h<15) {
colors = [0x381EA6, 0x381EA6];
alphas = [30, 20];
ratios = [0, 0xFF];
matrix = {a:500, b:0, c:0, d:0, e:200, f:0, g:200, h:200, i:1};
spreadMethod = "reflect";
interpolationMethod = "linearRGB";
focalPointRatio = 0.5;
lineStyle(1);
lineGradientStyle("linear", colors, alphas, ratios, matrix,
spreadMethod, interpolationMethod, focalPointRatio);
moveTo(0, 0 + (h * 50));
curveTo(500 + (h * 5), 100 + (h * 5), 200 + (h * 5), 0 + (h * 5));
curveTo(-400 + (h * 5), -170 + (h * 5), 1800 + (h * 100), 500 + (h * 100));
h++;
}
//bottom line------------------------------------------------------------
g=0;
while (g<15) {
colors = [0x381EA6, 0x381EA6];
alphas = [30, 20];
ratios = [0, 0xFF];
matrix = {a:500, b:0, c:0, d:0, e:200, f:0, g:200, h:200, i:1};
spreadMethod = "reflect";
interpolationMethod = "linearRGB";
focalPointRatio = 0.5;
lineStyle(1);
lineGradientStyle("linear", colors, alphas, ratios, matrix,
spreadMethod, interpolationMethod, focalPointRatio);
moveTo(0, 0 + (g * 50));
curveTo(500 + (g * 5), 100 + (g * 5), 200 + (g * 5), 0 + (g * 5));
curveTo(-400 + (g * 5), -170 + (g * 5), 1800 + (g * 100), 500 + (g * 100));
g++;
}
Flash glow effect
import flash.filters.*;
var glow:GlowFilter = new GlowFilter(0x00CCFF, 0.5, 2, 2, 4, 3);
MovieClip.filters = [glow];
var glow:GlowFilter = new GlowFilter(0x00CCFF, 0.5, 2, 2, 4, 3);
MovieClip.filters = [glow];
Flash stage auto resize
code:
function init() {
MovieClip._width = Stage.width;
MovieClip._height = Stage.height;
}
init();
var stageListener:Object = new Object();
stageListener.onResize = function() {
init();
}
Stage.addListener(stageListener);
function init() {
MovieClip._width = Stage.width;
MovieClip._height = Stage.height;
}
init();
var stageListener:Object = new Object();
stageListener.onResize = function() {
init();
}
Stage.addListener(stageListener);
Flash fade effect
code:
import mx.transitions.Tween;
import mx.transitions.easing.*;
new Tween(MovieClip, "_alpha", Strong.easeOut, 0, 100, 2, true);
note:
variable is MovieClip
import mx.transitions.Tween;
import mx.transitions.easing.*;
new Tween(MovieClip, "_alpha", Strong.easeOut, 0, 100, 2, true);
note:
variable is MovieClip
Flash movieClip randomizer
code:
function randomNo(high,low) {
generated = Math.floor(Math.random() * (high - low)) + low;
return generated;
}
_global.MovieClip_loader = function(MovieClip, url) {
var theLoader:MovieClipLoader = new MovieClipLoader();
theLoader.loadClip(Url, MovieClip);
var loaderListener:Object = new Object();
theLoader.addListener(loaderListener);
MovieClip.loader_txt.text = "0%";
loaderListener.onLoadProgress = function(_mc:MovieClip, loaded:Number, total:Number) {
MovieClip.loader_txt.text = Math.round((loaded/total) * 100) + "%";
}
loaderListener.onLoadComplete = function(_mc:MovieClip) {
MovieClip.loader_txt.text = "";
}
}
_global.MovieClip_randomizer = function(noOfClip) {
if (MovieClip_1.occupied == "") {
for (p=1;p<=noOfClip;p++) {
randomGenerated = randomNo(1,noOfClip);
if (p != 1 && p != noOfClip) {
while(eval("MovieClip_" + randomGenerated).occupied <> "") {
randomGenerated = randomNo(1,noOfClip);
}
}
if (p == noOfClip) {
for (r=1;r<=noOfClip;r++) {
if (eval("MovieClip_" + r).occupied == "") {
randomGenerated = r;
}
}
}
_global.MovieClip_loader("MovieClip_" + randomGenerated, swfName + p + ".swf");
}
}
}
note:
to use, e.g. _global.MovieClip_randomizer(3);
*if you have 3 mc: MovieClip_1, MovieClip_2, MovieClip_3
*the last clip will always be the last ;)
varibles are MovieClip, swfName, noOfClip
assuming that your movieClip names start from "MovieClip_"
function randomNo(high,low) {
generated = Math.floor(Math.random() * (high - low)) + low;
return generated;
}
_global.MovieClip_loader = function(MovieClip, url) {
var theLoader:MovieClipLoader = new MovieClipLoader();
theLoader.loadClip(Url, MovieClip);
var loaderListener:Object = new Object();
theLoader.addListener(loaderListener);
MovieClip.loader_txt.text = "0%";
loaderListener.onLoadProgress = function(_mc:MovieClip, loaded:Number, total:Number) {
MovieClip.loader_txt.text = Math.round((loaded/total) * 100) + "%";
}
loaderListener.onLoadComplete = function(_mc:MovieClip) {
MovieClip.loader_txt.text = "";
}
}
_global.MovieClip_randomizer = function(noOfClip) {
if (MovieClip_1.occupied == "") {
for (p=1;p<=noOfClip;p++) {
randomGenerated = randomNo(1,noOfClip);
if (p != 1 && p != noOfClip) {
while(eval("MovieClip_" + randomGenerated).occupied <> "") {
randomGenerated = randomNo(1,noOfClip);
}
}
if (p == noOfClip) {
for (r=1;r<=noOfClip;r++) {
if (eval("MovieClip_" + r).occupied == "") {
randomGenerated = r;
}
}
}
_global.MovieClip_loader("MovieClip_" + randomGenerated, swfName + p + ".swf");
}
}
}
note:
to use, e.g. _global.MovieClip_randomizer(3);
*if you have 3 mc: MovieClip_1, MovieClip_2, MovieClip_3
*the last clip will always be the last ;)
varibles are MovieClip, swfName, noOfClip
assuming that your movieClip names start from "MovieClip_"
Flash movieClip Loader
code:
var theLoader:MovieClipLoader = new MovieClipLoader();
theLoader.loadClip(Url, MovieClip);
var loaderListener:Object = new Object();
theLoader.addListener(loaderListener);
MovieClip.loader_txt.text = "0%";
loaderListener.onLoadProgress = function(_mc:MovieClip, loaded:Number, total:Number) {
MovieClip.loader_txt.text = Math.round((loaded/total) * 100) + "%";
}
loaderListener.onLoadComplete = function(_mc:MovieClip) {
MovieClip.loader_txt.text = "";
}
note:
variables are MovieClip and Url
var theLoader:MovieClipLoader = new MovieClipLoader();
theLoader.loadClip(Url, MovieClip);
var loaderListener:Object = new Object();
theLoader.addListener(loaderListener);
MovieClip.loader_txt.text = "0%";
loaderListener.onLoadProgress = function(_mc:MovieClip, loaded:Number, total:Number) {
MovieClip.loader_txt.text = Math.round((loaded/total) * 100) + "%";
}
loaderListener.onLoadComplete = function(_mc:MovieClip) {
MovieClip.loader_txt.text = "";
}
note:
variables are MovieClip and Url
Flash move dynamically
_global.moveXY = function(targetx,targety,speed) {
goAnimate = function() {
this._x += (targetx - this._x)/speed;
this._y += (targety - this._y)/speed;
if((Math.abs(this._x - targetx) < 0.5) && (Math.abs(this._y - targety) < 0.5)) {
delete this.onEnterFrame;
}
}
this.onEnterFrame = goAnimate;
}
to use the script,
_global.moveXY.call(movieClip name, x, y, speed);
Flash random number function
function randomNo(high,low) {
generated = Math.floor(Math.random() * (high - low)) + low;
return generated;
}
note:
high = the highest number to random
low = the lowest number to random
generated = Math.floor(Math.random() * (high - low)) + low;
return generated;
}
note:
high = the highest number to random
low = the lowest number to random
Subscribe to:
Posts (Atom)